Mesh Generation
Note
Remember that ADflow is a 3D finite volume solver. Therefore, 3D meshes are needed even for 2D problems such as airfoil simulations. To do this, we generate a 3D mesh which is one cell wide, and apply symmetry boundary conditions on those two faces.
In this tutorial, we will use pyHyp to generate a 3D mesh in CGNS format.
The coordinates for the NACA0012 airfoil are in the file n0012.dat
.
Coordinates for most airfoils can be obtained from the UIUC Data site.
The initial mesh (n0012.dat
) can be visualized in Tecplot.
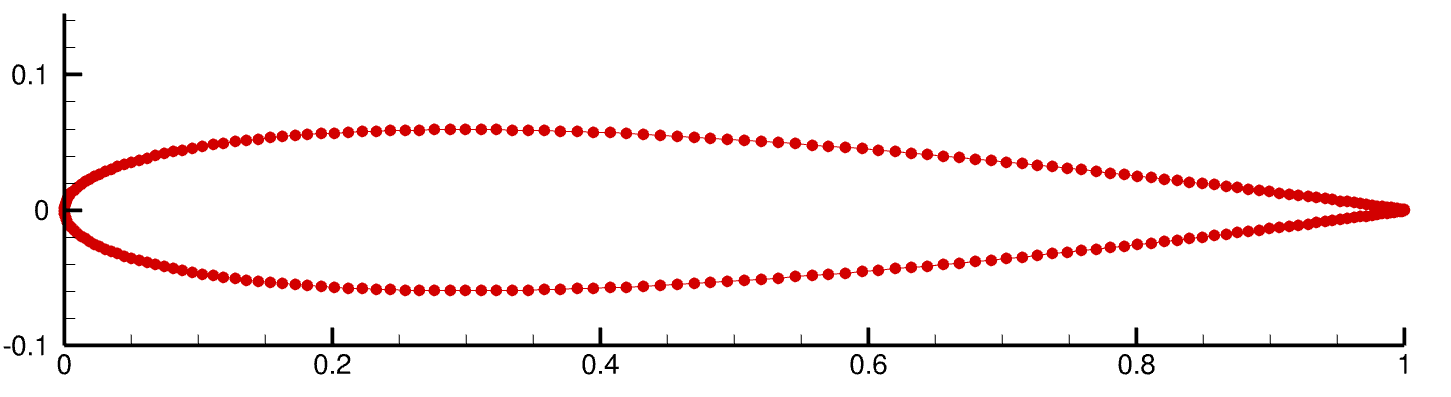
Navigate to the directory airfoilopt/mesh
in your tutorial folder. Copy the airfoil data from the tutorial
directory:
cp ../../../tutorial/airfoilopt/mesh/n0012.dat .
Create the following empty runscript in the current directory.
genMesh.py
pyHyp runscript
Import the pyHyp libraries and numpy.
import numpy as np
from pyhyp import pyHyp
Surface Mesh Generation
data = np.loadtxt("n0012.dat")
x = data[:, 0].copy()
y = data[:, 1].copy()
ndim = x.shape[0]
airfoil3d = np.zeros((ndim, 2, 3))
for j in range(2):
airfoil3d[:, j, 0] = x[:]
airfoil3d[:, j, 1] = y[:]
# set the z value on two sides to 0 and 1
airfoil3d[:, 0, 2] = 0.0
airfoil3d[:, 1, 2] = 1.0
# write out plot3d
P3D_fname = "n0012.xyz"
with open(P3D_fname, "w") as p3d:
p3d.write(str(1) + "\n")
p3d.write(str(ndim) + " " + str(2) + " " + str(1) + "\n")
for ell in range(3):
for j in range(2):
for i in range(ndim):
p3d.write("%.15f\n" % (airfoil3d[i, j, ell]))
pyHyp requires a surface mesh input before it can create a 3D mesh.
An “extruded” surface mesh can be created using the code above, which produces a PLOT3D file with extension .xyz
.
This meshes only the airfoil surface, and is used as the input file for pyHyp, which marches the existing mesh to the farfield.
By performing this intermediate step, the volume mesh generation is faster and higher-quality.
The intermediate mesh (new.xyz
) can be visualized in Tecplot.
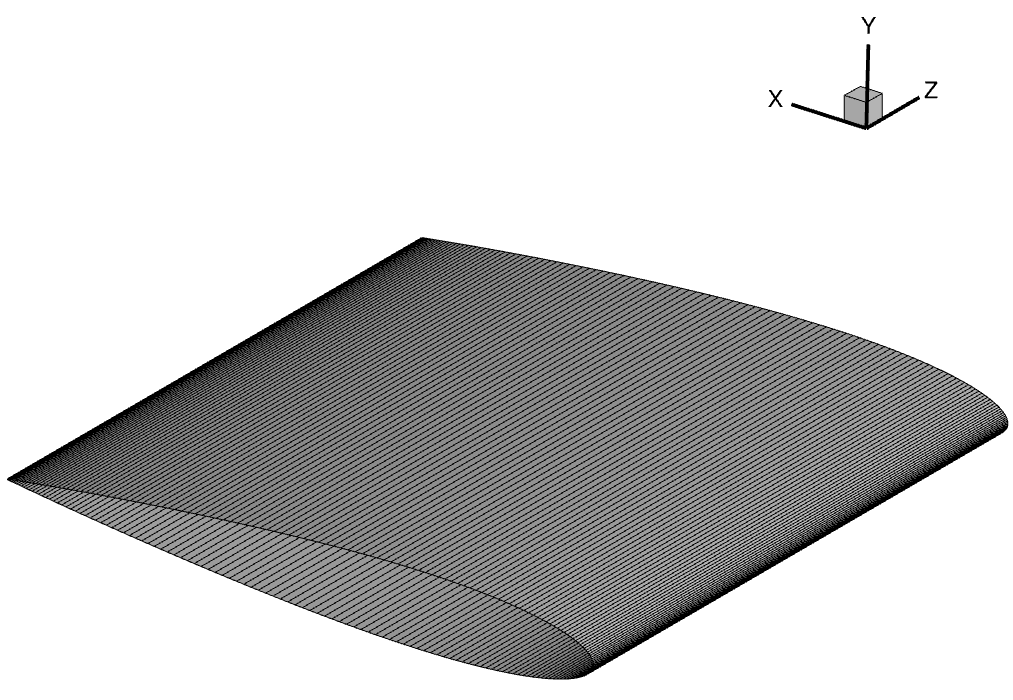
Options
options = {
# ---------------------------
# Input Parameters
# ---------------------------
"inputFile": P3D_fname,
"unattachedEdgesAreSymmetry": False,
"outerFaceBC": "farfield",
"autoConnect": True,
"BC": {1: {"jLow": "zSymm", "jHigh": "zSymm"}},
"families": "wall",
General Options
inputFile
Name of the surface mesh file.
unattachedEdgesAreSymmetry
Tells pyHyp to automatically apply symmetry boundary conditions to any unattached edges (those that do not interface with another block).
outerFaceBC
Tells pyHyp which boundary condition to apply to the outermost face of the extruded mesh. Note that we do not set the inlet or outlet boundaries seperately because they are automatically handled in ADflow consistently with the free stream flow direction.
BC
Tells pyHyp that, since it is a 2D problem, both sides of the domain
jLow
andjHigh
are set to be symmetry boundary conditions. The input surface is automatically assigned to be a wall boundary.families
Name given to wall surfaces. If a dictionary is submitted, each wall patch can have a different name. This can help the user to apply certain operations to specific wall patches in ADflow.
# ---------------------------
# Grid Parameters
# ---------------------------
"N": 129,
"s0": 3e-6,
"marchDist": 100.0,
}
Grid Parameters
N
Number of nodes in off-wall direction. If multigrid will be used this number should be 2m-1(n+1), where m is the number of multigrid levels and n is the number of layers on the coarsest mesh.
s0
Thickness of first off-wall cell layer.
marchDist
Distance of the far-field.
Running pyHyp and Writing to File
The following three lines of code extrude the surface mesh and write the resulting volume mesh to a .cgns
file.
hyp = pyHyp(options=options)
hyp.run()
hyp.writeCGNS("n0012.cgns")
Run it yourself!
You can now run the python file with the command:
python genMesh.py
The generated airfoil mesh should look like the following.
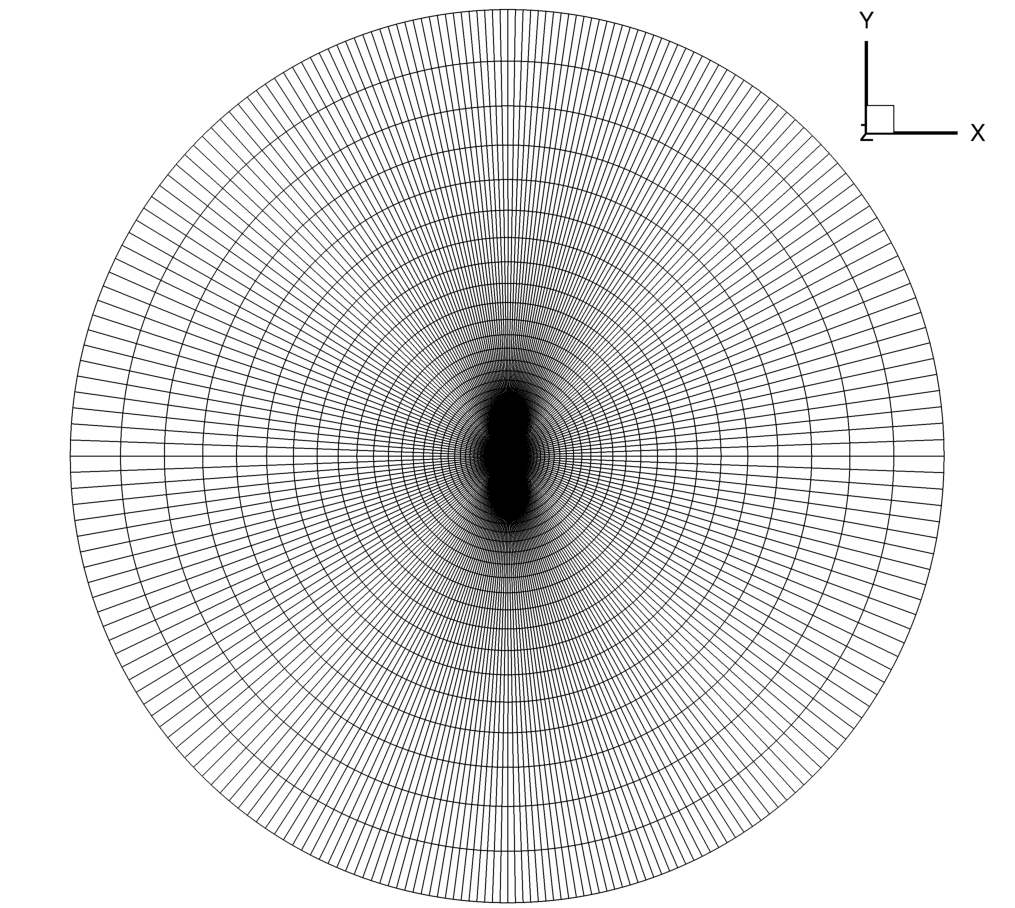
with a zoomed-in view:
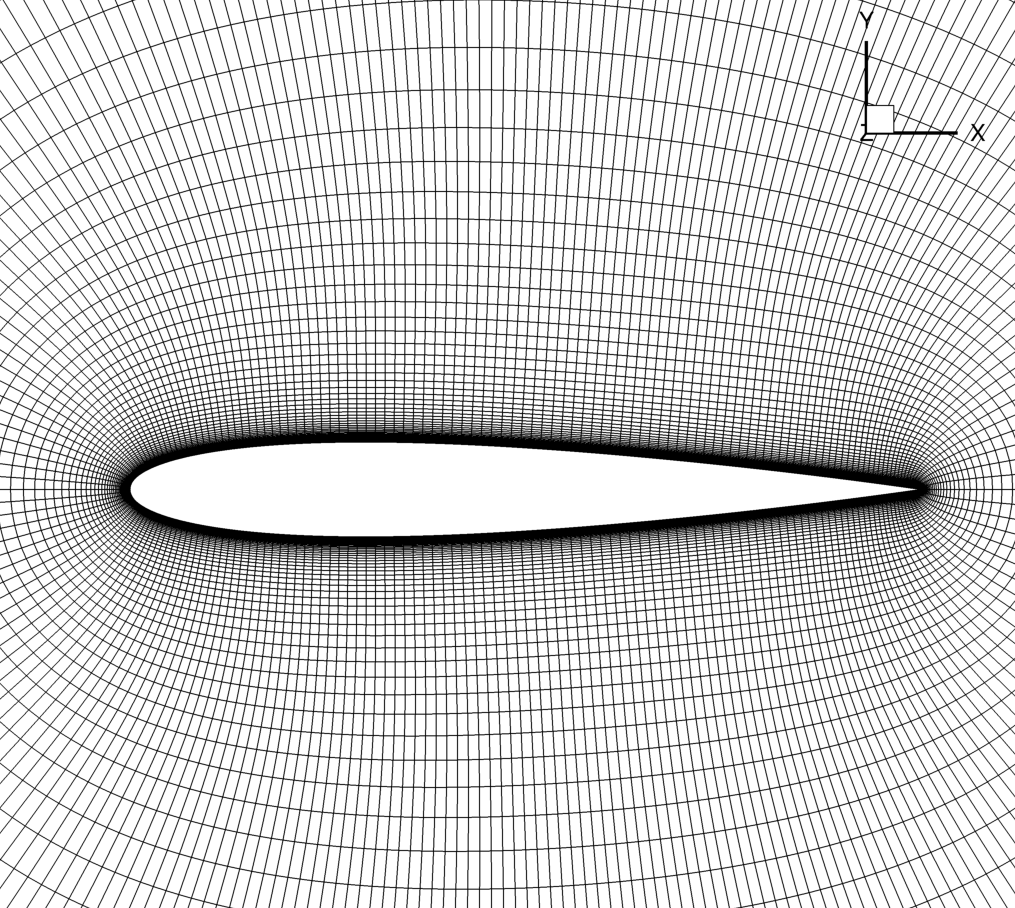