Aerodynamic Analysis
For the rest of this tutorial, we will use INPUT/cfd/dlr-f6_vol.cgns
as the CFD volume mesh.
This is an overset mesh that includes the fuselage, wing, and wing-fuselage collar meshes.
We start with running aerodynamic analysis.
The aerodynamic analysis script for a wing-fuselage configuration is largely the same as for a wing, as shown in aero_run.py
.
import os
from mpi4py import MPI
from SETUP import setup_aeroproblem, setup_adflow
# Setup I/O
comm = MPI.COMM_WORLD
gridFile = "INPUT/cfd/dlr-f6_vol.cgns"
outDir = "OUTPUT/analysis"
# Create output directory if it does not exist
if comm.rank == 0:
if not os.path.exists(outDir):
os.makedirs(outDir)
# Set up ADflow
CFDSolver = setup_adflow.setup(comm, gridFile, outDir)[0]
# Set up AeroProblem
ap = setup_aeroproblem.setup()
# Run ADflow
CFDSolver(ap)
# Evaluate functions
funcs = {}
CFDSolver.evalFunctions(ap, funcs)
# Print the evaluated functions
CFDSolver.pp(funcs)
One difference is that we use the cutCallback
option when setting up ADflow.
This ensures that cells on the negative side of the y-axis are blanked.
# Define function to blank cells behind symmetry plane
def cutCallback(xCen, CGNSZoneNameIDs, cellIDs, flags):
flags[xCen[:, 1] < 0] = 1
The full setup scripts are in SETUP/setup_aeroproblem.py
and SETUP/setup_adflow.py
.
Surface Families
For multi-component geometries, it is often convenient to define custom surface families in ADflow.
In this example, we create a wing_full
family that combines the wing and wing collar meshes.
The resulting surface family comprises the entire wing surface.
This is useful for plotting the lift distribution and slices along the wing.
# Add wing family group
CFDSolver.addFamilyGroup(
"wing_full",
[
"intersection_0",
"wing",
],
)
# Add wing and total lift distribution
CFDSolver.addLiftDistribution(200, "y", groupName="wing_full")
CFDSolver.addLiftDistribution(200, "y")
# Add fuselage slice
CFDSolver.addSlices("y", 0.0001)
# Add wing slices
CFDSolver.addSlices("y", np.linspace(0.074, 0.55, 10), groupName="wing_full")
If we do not specify a groupName
, ADflow will use the allWalls
family by default.
As the name suggests, this is a family that includes all wall surfaces.
For the fuselage slice, using allWalls
is fine because we are taking a slice close to the symmetry plane where only the fuselage surface is present.
For the wing slices, using allWalls
can result in a slice near the wing root that contains both the wing and fuselage, as shown below.
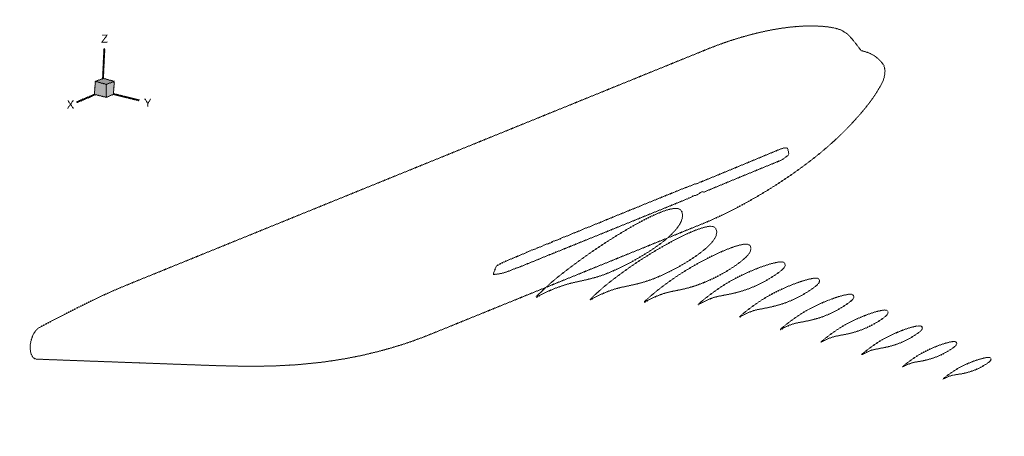
Slices using only allWalls
Using the custom wing_full
family, we obtain a slice at the wing root that contains only the wing.
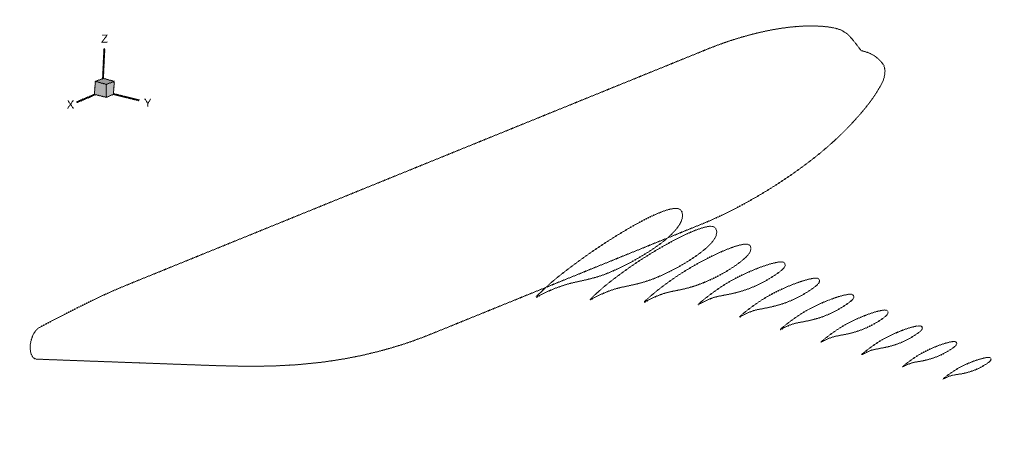
Slices using wing_full
for the wing slices